Billion-Dollar Mistake and How Kotlin Solved It
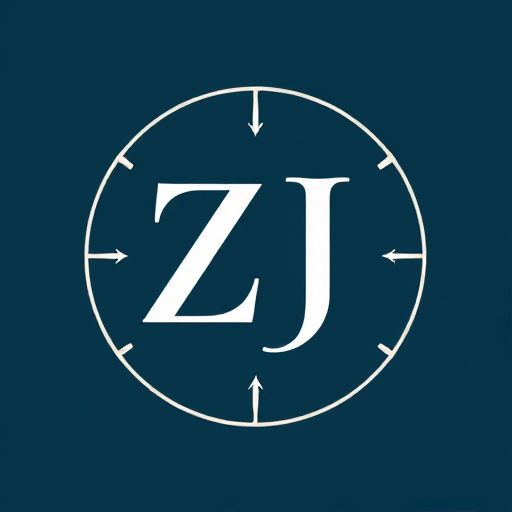
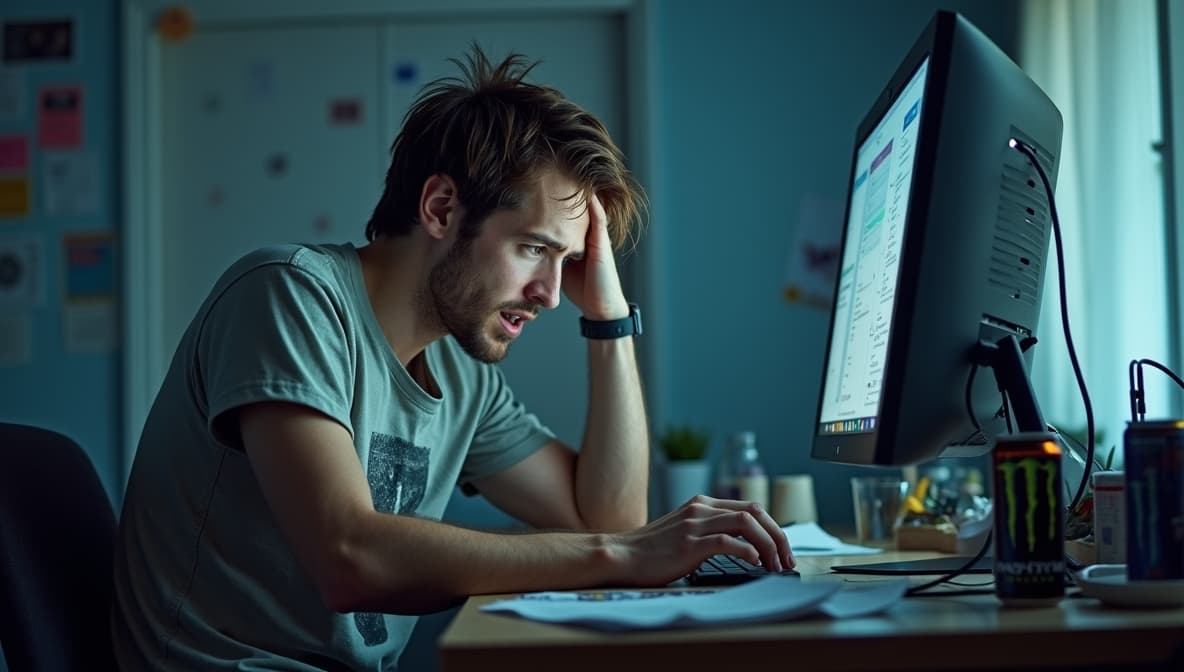
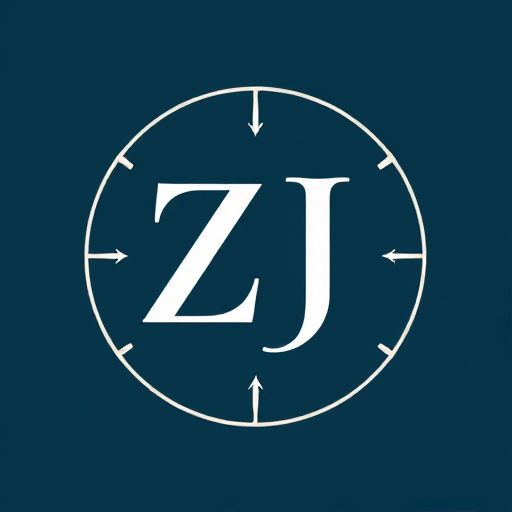
The "billion-dollar mistake" refers to null references, a concept introduced by Tony Hoare in 1965. Null references can cause errors like crashes and bugs when used incorrectly, and fixing these issues is costly.
Why Accessing Null References Is a Problem
Accessing null references or their properties is an issue because it leads to a NullPointerException (or similar errors in other languages). These errors occur when a program tries to use an object that has not been initialized or explicitly set to null.
This disrupts program execution and often causes crashes. Debugging such issues can be time-consuming and expensive, especially in large codebases. Ensuring that a variable is not null before using it is a critical practice in programming.
Example in Java:
public class NullReferenceExample {
public static void main(String[] args) {
String name = null; // Null reference
try {
// This will throw a NullPointerException
System.out.println(name.length());
} catch (NullPointerException e) {
System.out.println("Caught a NullPointerException!");
}
}
}
Kotlin's Solution to Null References
Kotlin addresses the "billion-dollar mistake" by introducing nullable and non-nullable types directly into the type system. This helps developers catch potential null pointer issues at compile time instead of runtime.
Example in Kotlin:
fun main() {
val name: String? = null // Nullable type
// Safe call operator to avoid NullPointerException
println(name?.length) // Prints: null
val nonNullName: String = "Kotlin"
println(nonNullName.length) // Prints: 6
// Uncommenting the line below would cause a compile-time error:
// println(name.length)
// Elvis operator for default values
val length = name?.length ?: 0
println("Length: $length") // Prints: Length: 0
}
In this example:
- String? declares a nullable type, allowing the variable to hold null.
- The safe call operator (``) prevents a NullPointerException by checking if the value is null before accessing it.
- The Elvis operator (``) provides a default value if the nullable type is null. It acts like a "fallback" mechanism: if the expression on the left of the Elvis operator evaluates to null, the value on the right is used instead.
More About the Elvis Operator:
The Elvis operator is named after the resemblance of its symbol ?: to an emoji or face with a swoop of hair, reminiscent of Elvis Presley. It simplifies null-checking code that would otherwise require more verbose constructs like:
val length = if (name != null) name.length else 0
With the Elvis operator, the same logic is written as:
val length = name?.length ?: 0